
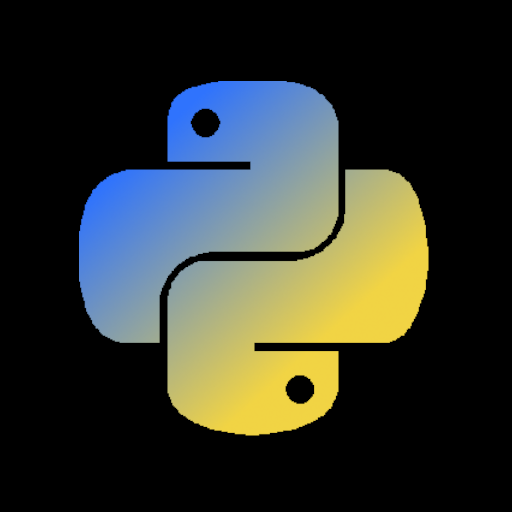
No problems. Learning a new concept is not stupid. So you are familiar with C. In C term, you are likely to do something like this:
int a[10] = {0}; // Just imagine this is 0,1,2,etc...
int b[10] = {0};
for (int i=0; i < 10; i++) {
b[i] = a[i]*2;
}
A 1 to 1 correspondent might looks like ths:
a = range(10) # 0,1,2,etc...
b = []
for x in a:
b.append(x*2)
However in python, you can then simplify to this:
a = range(10) # Same as before, 0,1,2,etc...
b = [x*2 for x in a]
# This is also works
b = [x*2 for x in [0,1,2,...]]
Remember that list comprehension is used to make a new list, not just iteration. If you want to do something other than making a list from another list, it is better to use iteration. List comprehension is just “syntactic sugar” so to speak. The concept comes from functional programming paradigm.
Good
Edit: Oh shit nvm. It still requires dedicated HW (FPGA). This is no different than say, an NPU. But to be fair, they also said the researcher tested the model on traditional GPU too and reduce memory consumption.